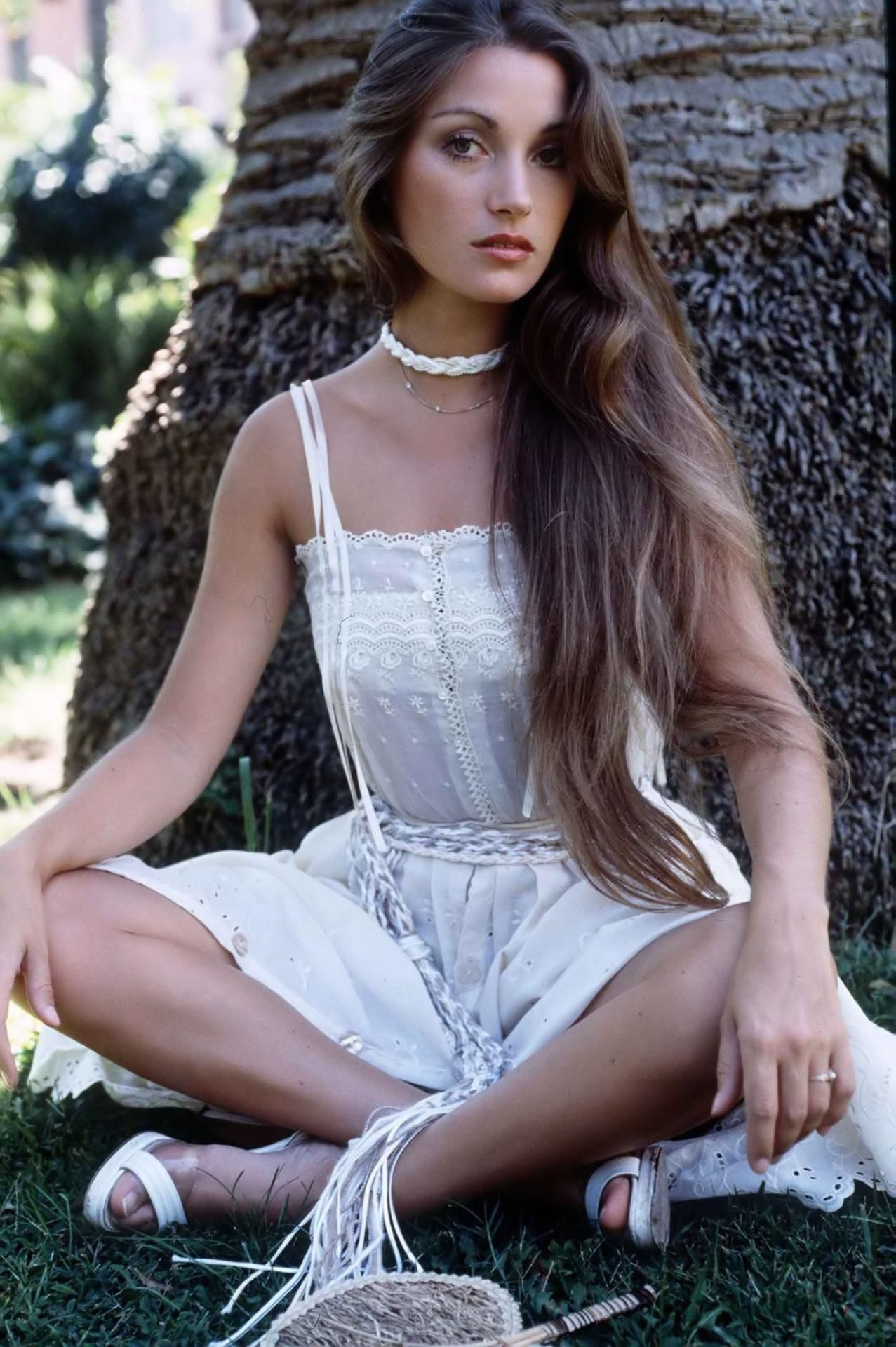
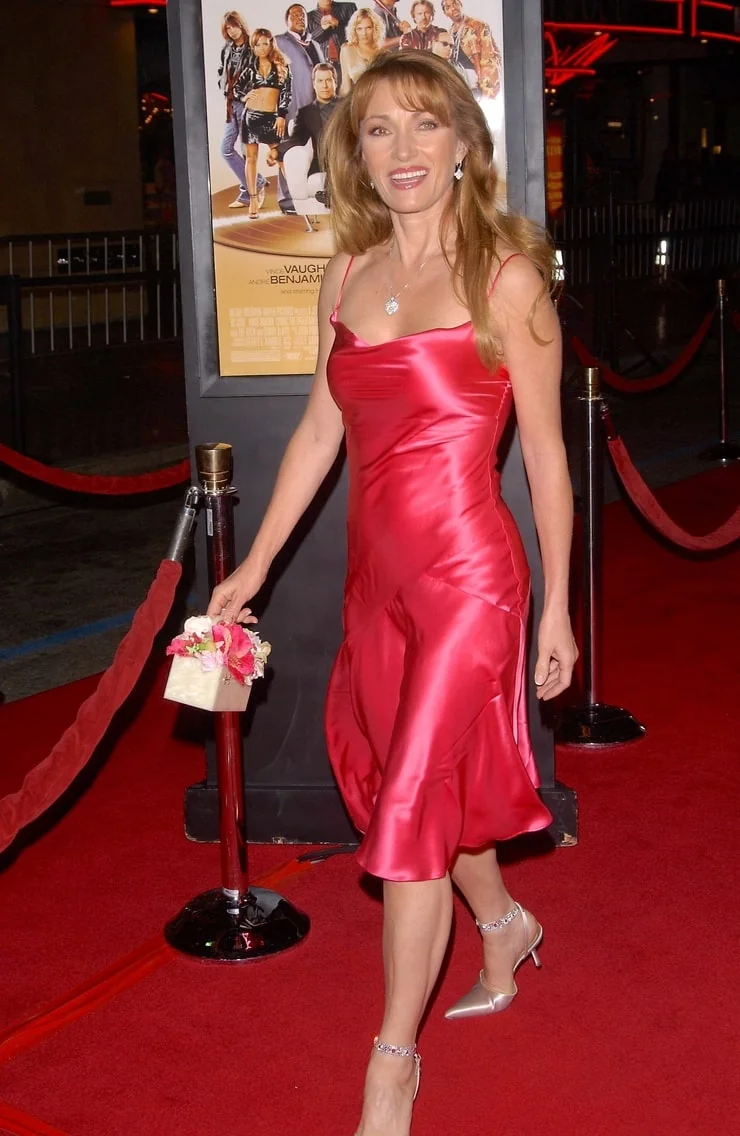
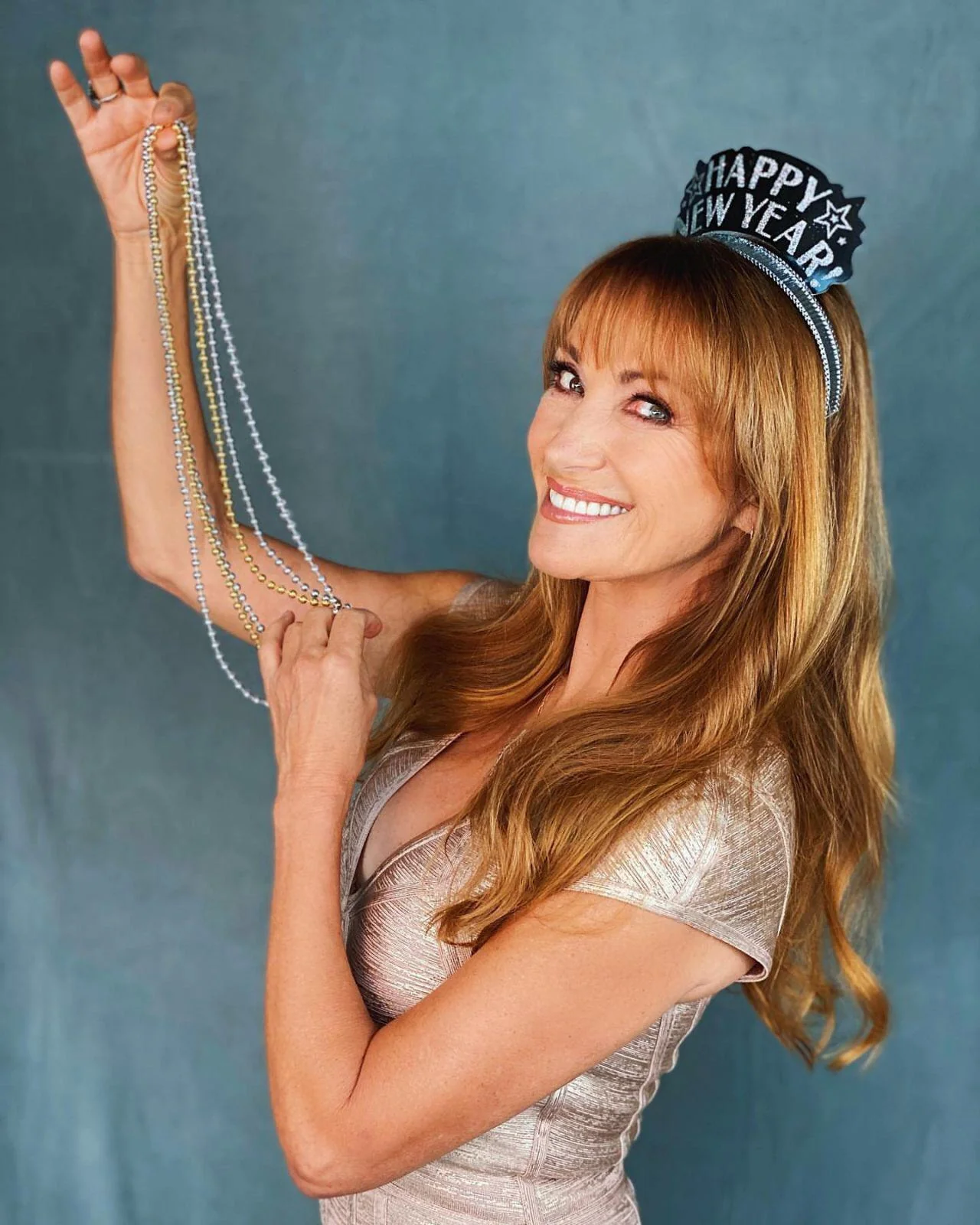
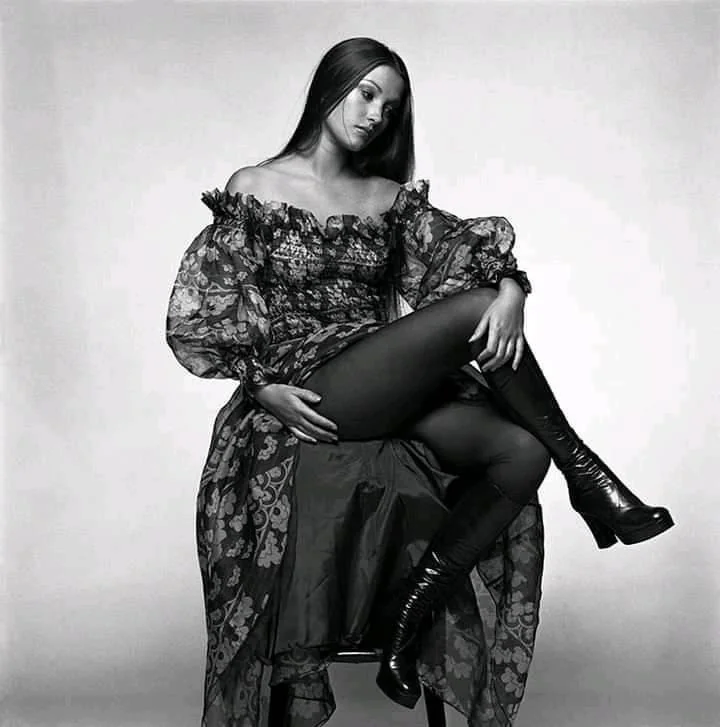
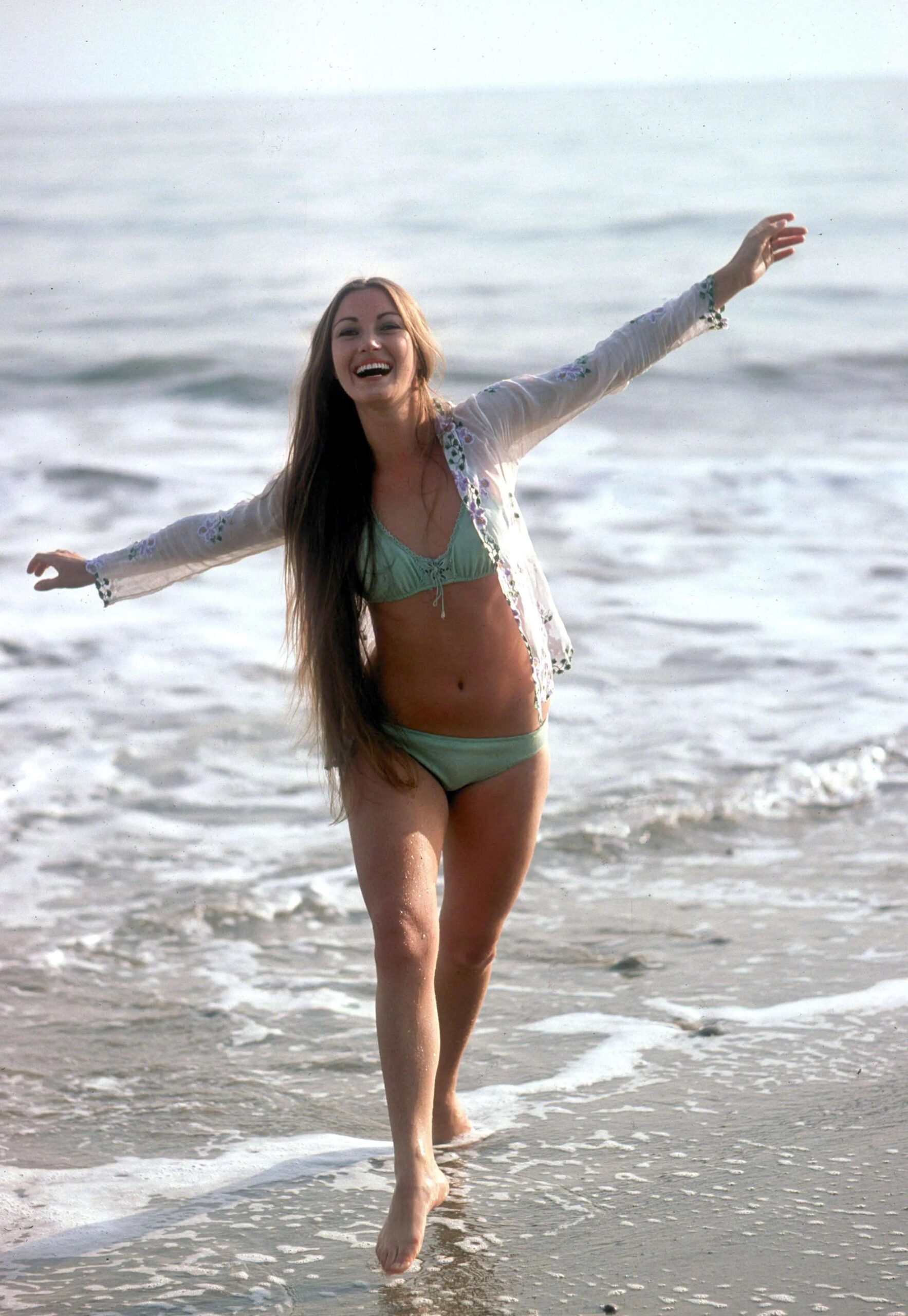
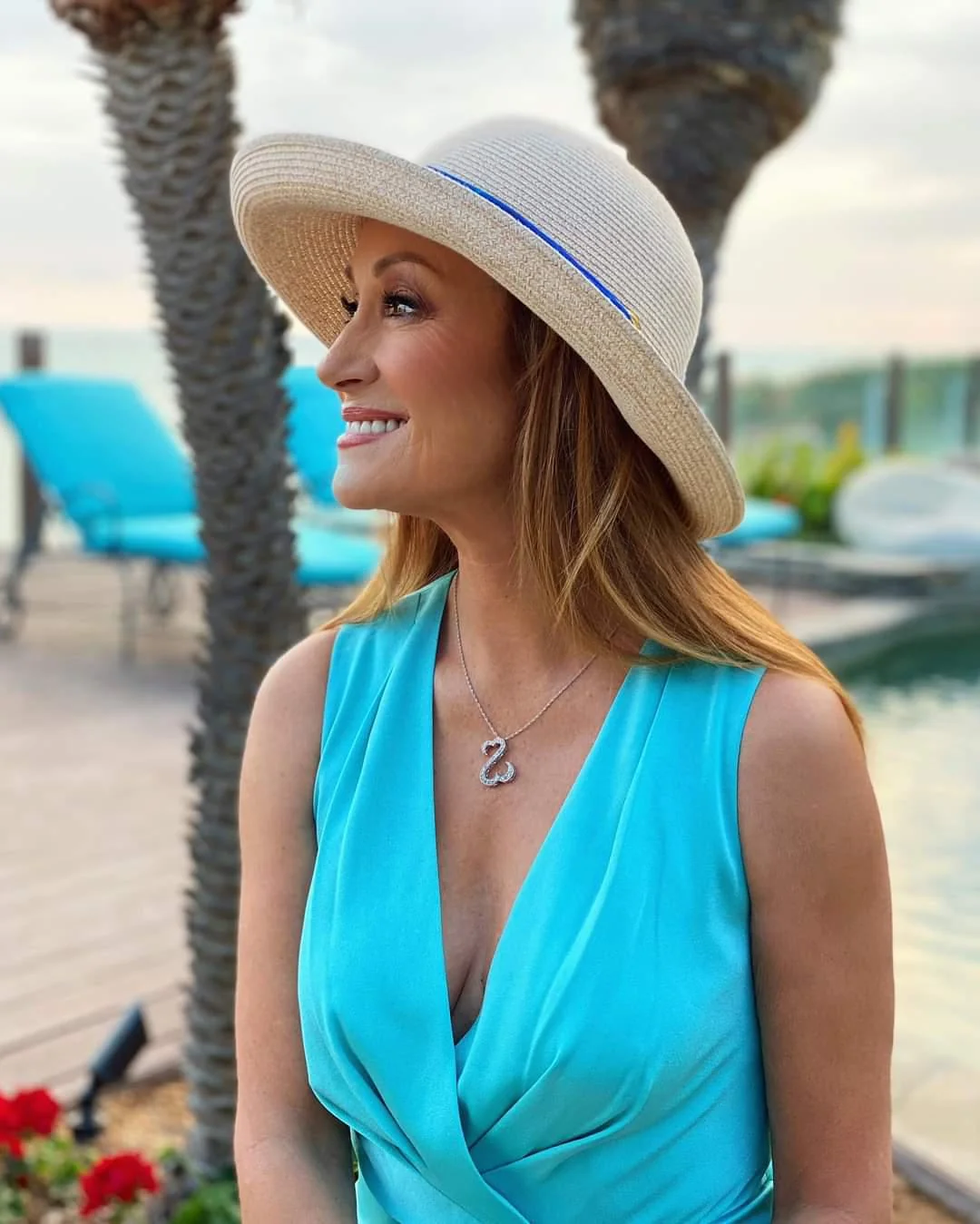
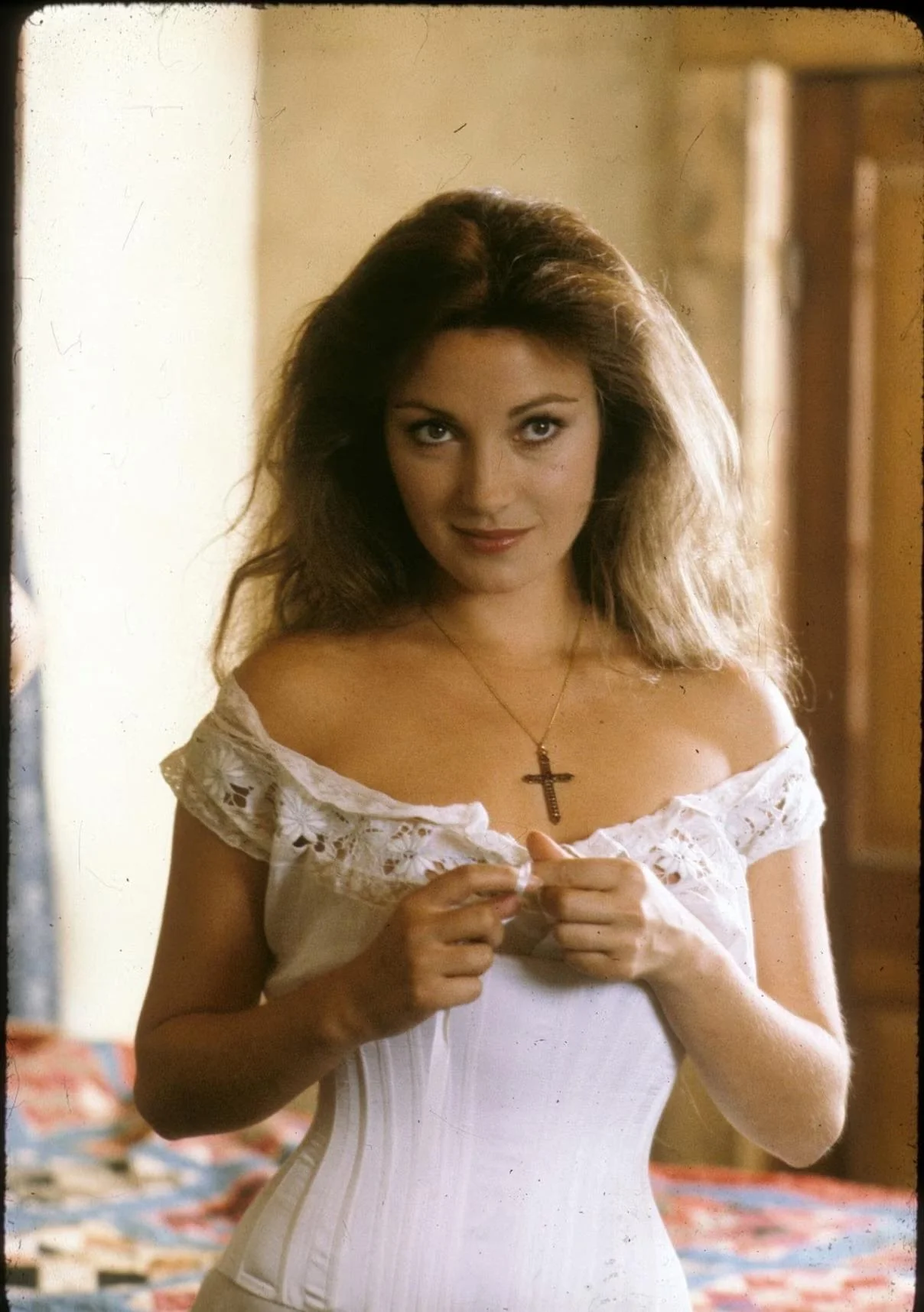
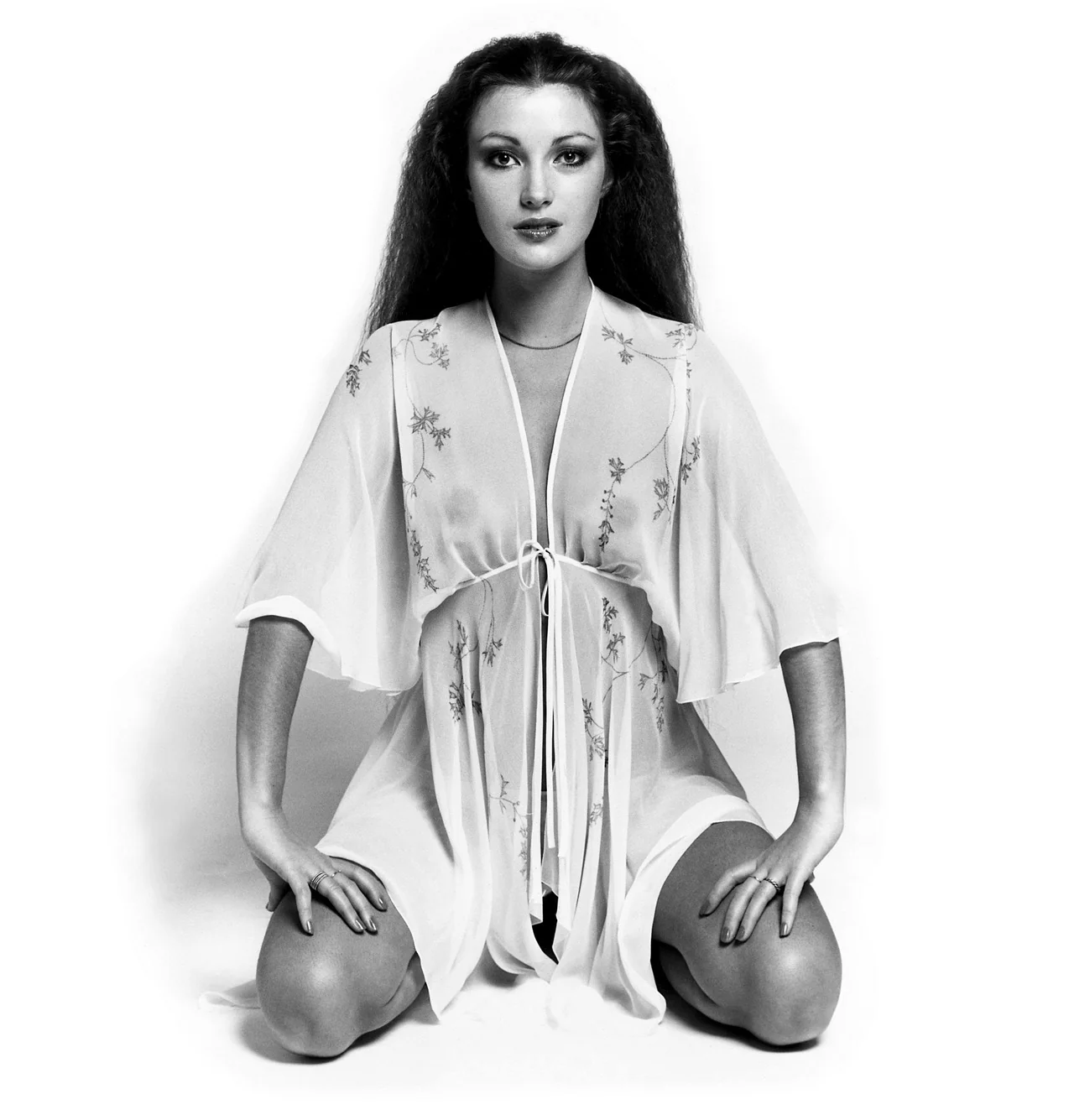
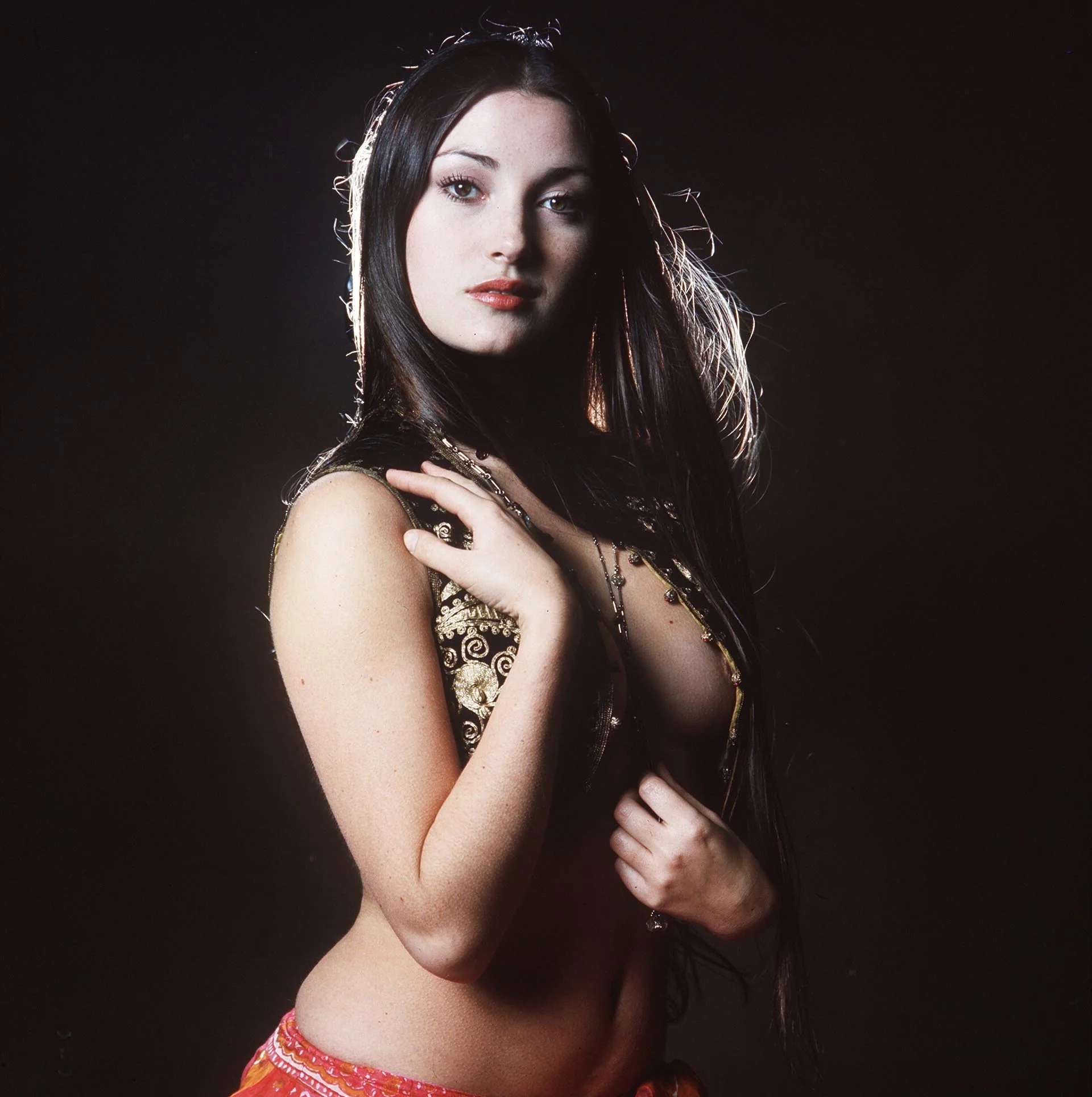
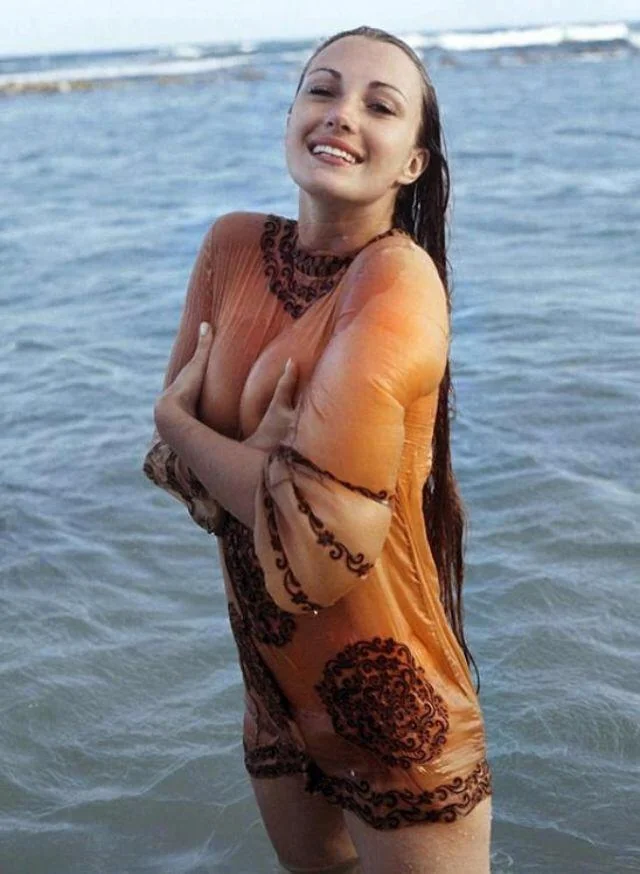
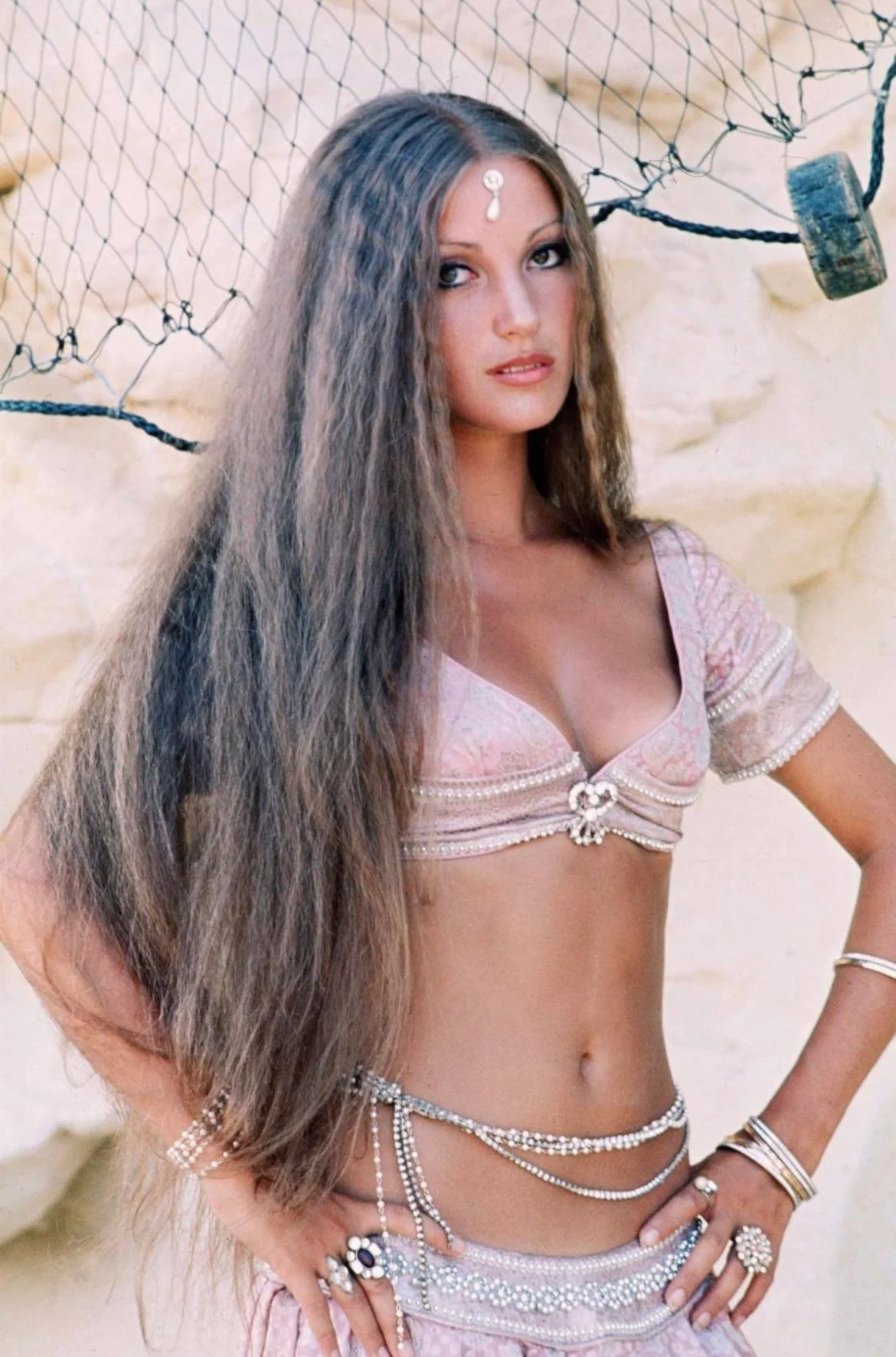
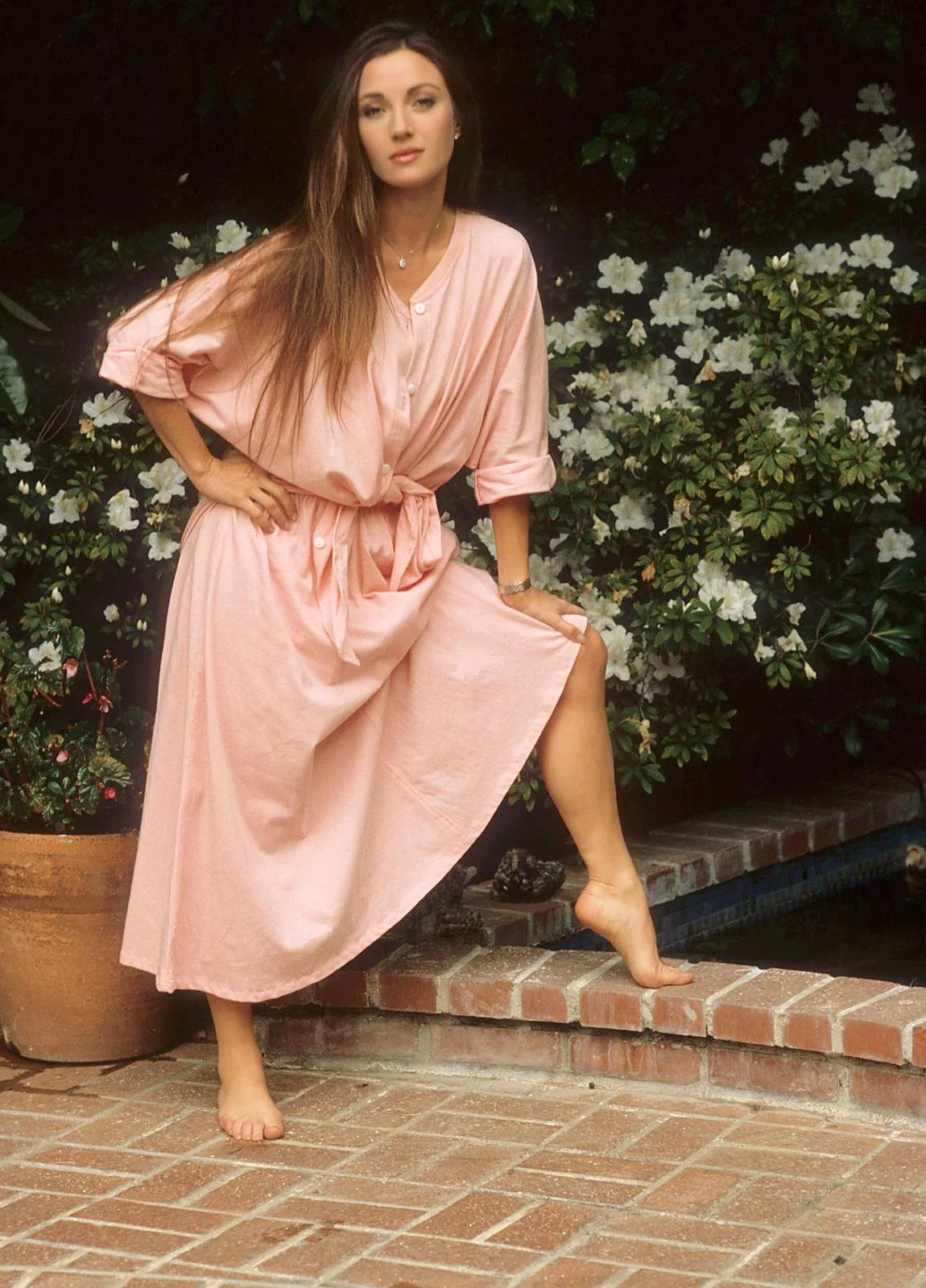
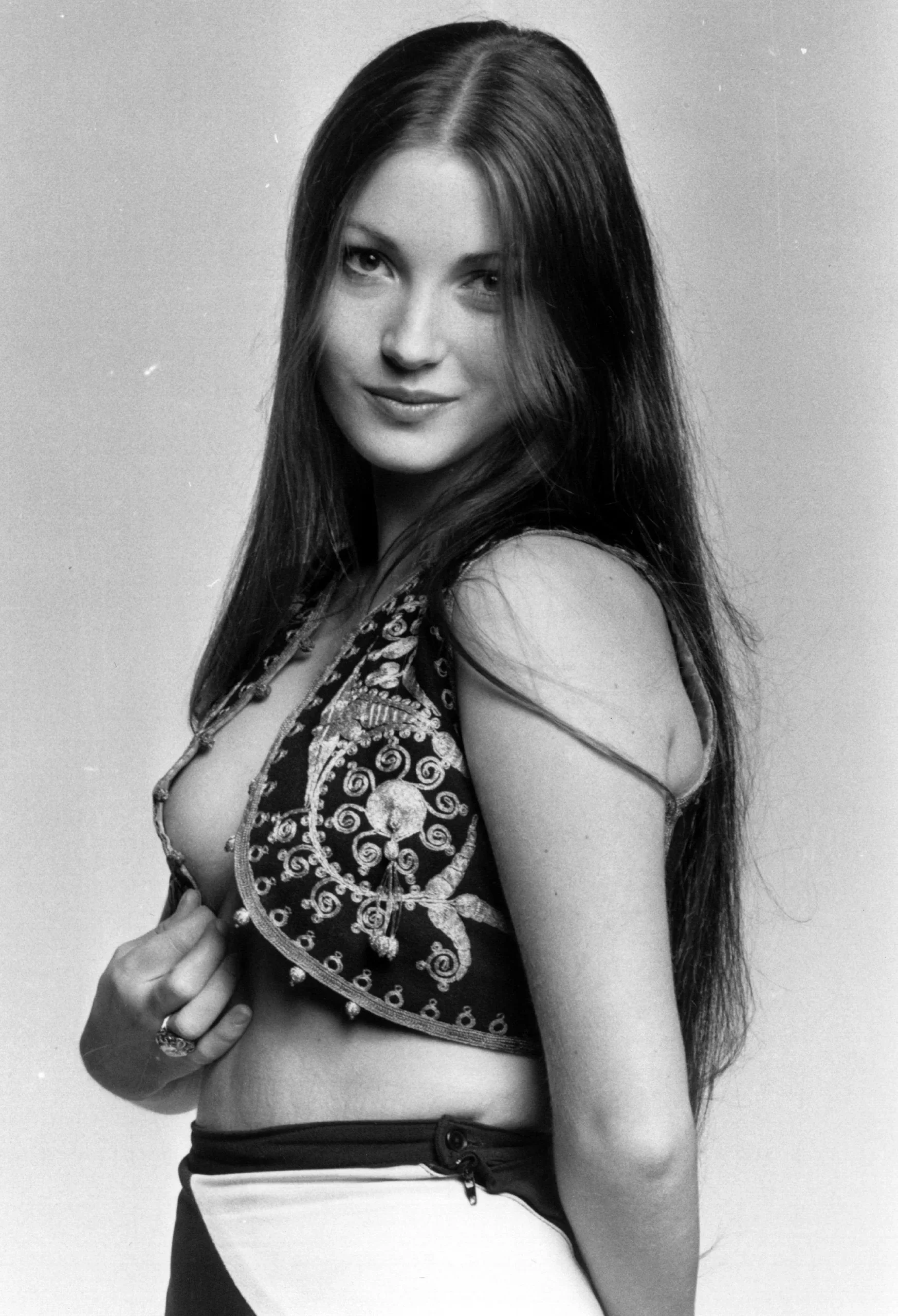
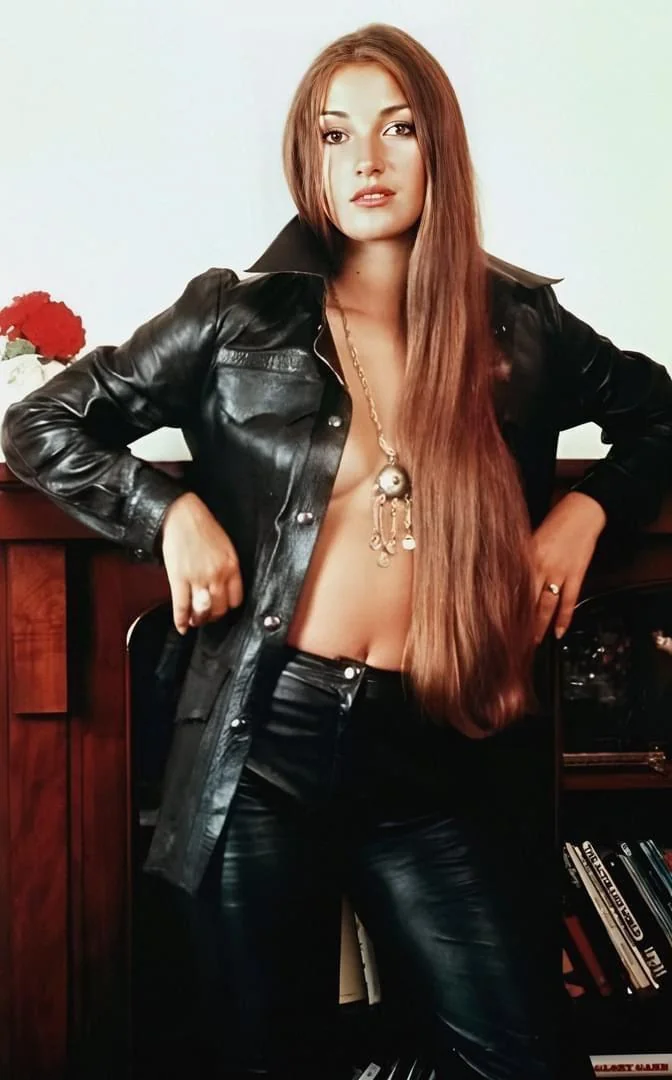
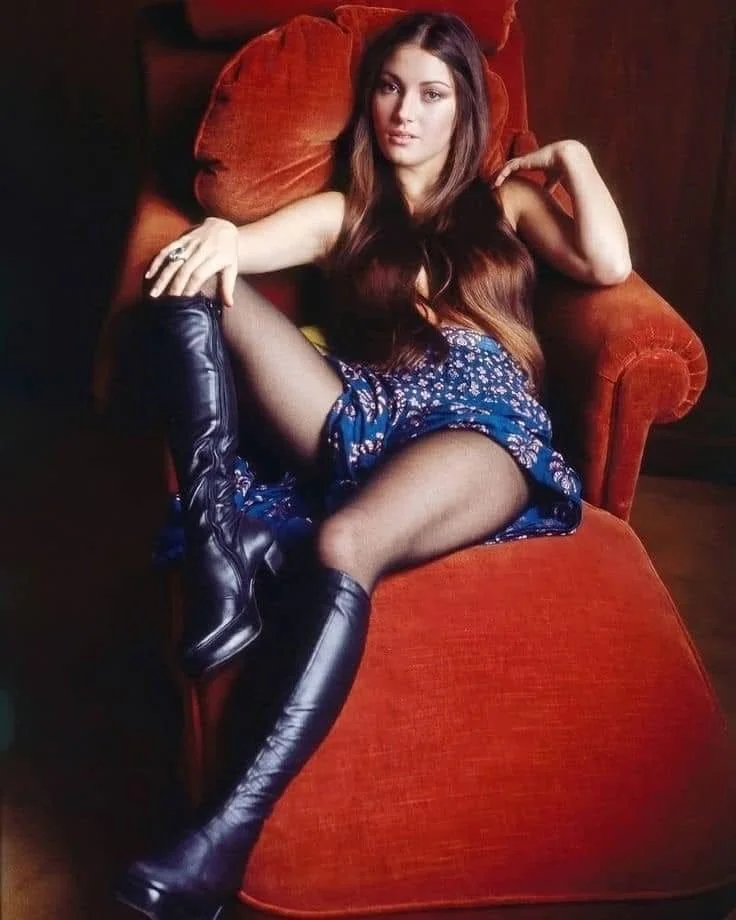
Introduction: What are data structures and why are they important?
Data structures are the backbone of computer science. They help organize and manage data efficiently, making it easier for programmers to access and manipulate information. But what exactly are data structures? Essentially, they are specific ways to store and arrange data in a computer so that we can use it effectively.
Understanding data structures is vital for anyone involved in programming or software development. Why? Because the choice of a suitable data structure can significantly affect performance—even small changes can lead to faster execution times or reduced memory usage. Whether you’re building an application from scratch or optimizing existing code, knowing your way around various data structures will empower you to make smarter decisions.
Let’s dive into the fascinating world of data structures and explore their types, advantages, applications, and how you can choose the best one for your needs!
Types of Data Structures:
Data structures come in various forms, each serving distinct purposes. One of the most fundamental is the array. Arrays store elements in contiguous memory locations, allowing for quick access by index. This makes them efficient for tasks that require frequent read operations.
Next up is the linked list. Unlike arrays, linked lists consist of nodes connected by pointers. They are dynamic in nature and allow easy insertion or deletion of elements without needing to shift others around.
Stacks operate on a last-in, first-out (LIFO) principle. Think of it as a stack of plates; you add and remove items from the top only. This structure is useful for scenarios like undo features in applications.
Queues function on a first-in, first-out (FIFO) basis, resembling lines at a movie theater. Items enter at one end and exit from another, making queues essential for scheduling tasks efficiently across systems.
– Array
An array is a fundamental data structure that stores elements in a contiguous block of memory. This allows for fast access and manipulation, making it one of the most widely used structures in programming.
Arrays can hold multiple values under a single name, with each element identified by an index. For example, in a list of student grades, you could easily retrieve the grade for any student using their position in the array.
One of the key benefits of arrays is their efficiency. Accessing an element by its index takes constant time, O(1), which makes operations like searching or updating quick and straightforward.
However, arrays have limitations too. Their size must be defined at creation; resizing requires creating a new array and copying over existing elements. This fixed nature can pose challenges when dealing with dynamic datasets that grow or shrink frequently.
– Linked List
A linked list is a dynamic data structure that consists of nodes. Each node contains two components: the data and a reference (or pointer) to the next node in the sequence. This design allows for efficient insertion and deletion operations since elements can be added or removed without reorganizing the entire structure.
Unlike arrays, which have fixed sizes, linked lists can grow and shrink as needed. They are particularly useful for applications where frequent additions or deletions occur.
There are several types of linked lists—singly linked, doubly linked, and circular. Each type offers its own advantages depending on specific use cases. For instance, doubly linked lists allow traversal in both directions but require more memory due to an additional pointer.
In scenarios where memory allocation needs flexibility, a linked list shines as an excellent choice for managing collections of items dynamically.
– Stack
A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. Imagine it like a pile of plates; the last plate placed on top is the first one you’ll remove. This simplicity makes stacks particularly useful in various applications.
Operations on a stack are straightforward: you can push an item onto it or pop an item off. These operations ensure quick access to the most recently added elements, which can be vital in scenarios such as function calls and undo mechanisms in software.
Stacks also allow for efficient memory management during program execution. They help keep track of active subroutines, making them essential for recursion and backtracking algorithms.
Understanding how stacks work opens up new possibilities for solving complex problems with elegant solutions. Their structure may seem basic, but their utility is profound across programming languages and systems.
– Queue
A queue is a fundamental data structure that operates on the principle of First-In-First-Out (FIFO). This means that elements added first will be the ones to be removed first. Think of it like people waiting in line at a coffee shop; the person who arrives first gets served before anyone else.
Queues are widely used in various applications, particularly where order matters. For example, they play a critical role in managing tasks in computer processes and handling requests for resources.
Implementation can vary from simple arrays to more complex linked lists. Each method has its benefits depending on specific use cases, such as memory efficiency or speed of access.
They also support essential operations: enqueue (adding an item) and dequeue (removing an item), making them straightforward yet powerful tools for developers. The simplicity of queues belies their usefulness across numerous fields and technologies.
Comparison of Data Structures: Advantages and Disadvantages
When comparing data structures, it’s essential to weigh their advantages and disadvantages based on specific needs.
Arrays are straightforward. They offer fast access to elements due to their contiguous memory allocation. However, resizing them is a hassle since they require reallocation.
Linked lists shine in dynamic memory management. Inserting or deleting nodes can be done efficiently without shifting other elements. Yet, they incur extra overhead for storing pointers and may lead to increased space usage.
Stacks follow a last-in, first-out (LIFO) principle. This makes them ideal for tasks like reversing strings or parsing expressions. Their downside? Limited accessibility; you can only interact with the top element directly.
Queues operate on a first-in, first-out (FIFO) basis, perfect for scheduling tasks and managing requests. But like stacks, accessing arbitrary elements isn’t possible without dequeuing others first.
Real-world Applications of Data Structures
Data structures play a crucial role in various real-world applications. In web development, they help manage user data efficiently. For instance, arrays are often used to store lists of items, such as product catalogs for e-commerce sites.
In social media platforms, linked lists facilitate the dynamic management of posts and comments. They allow quick updates and deletions without significant overhead.
Stacks are vital in programming languages for handling function calls. When you open multiple tabs in a browser, stacks keep track of your history seamlessly.
Queues find their place in customer service systems where requests need to be processed in order. This ensures fair treatment for all users seeking assistance.
Moreover, databases utilize sophisticated data structures to enable efficient querying and storage retrieval. Understanding these applications can enhance system performance across various industries.
Choosing the Best Data Structure for Your Needs
Choosing the right data structure is crucial for your project’s success. It depends on what you need to achieve. Consider factors such as speed, memory usage, and ease of implementation.
For quick access to elements, arrays might be ideal due to their simple index-based system. However, if you anticipate frequent insertions or deletions, a linked list could serve you better with its dynamic nature.
Stacks are perfect for scenarios requiring last-in-first-out processing like undo features in software. On the other hand, queues excel when tasks must follow a first-come-first-served order—think printing jobs lined up in an office.
Always assess your specific use case before making a decision. Testing different structures can also reveal which one performs best under your unique conditions. Understanding these nuances will help streamline development and enhance performance significantly.
Tips for Implementing Data Structures Effectively
When implementing data structures, clarity is key. Start by clearly defining the problem you’re trying to solve. This helps in selecting the most suitable structure.
It’s important to understand each data structure’s strengths and weaknesses. For instance, arrays offer fast access but lack flexibility. On the other hand, linked lists provide dynamic sizing.
Consider scalability early on. As your application grows, so will its data requirements. Choose a data structure that can handle future demands without major overhauls.
Always prioritize readability in your code. Well-named variables and clear comments enhance maintainability for you and others who might work with your code later.
Testing is vital before deployment. Implement thorough tests to ensure that the chosen structure performs as expected under different scenarios.
Stay updated with emerging trends in technology. New algorithms or structures could revolutionize how you approach problems down the road.
Future Developments in the Field of Data Structures
The future of data structures is poised for exciting advancements. As technology evolves, so do the needs for more efficient ways to store and manage data.
One significant trend is the integration of machine learning with data structures. Smart algorithms will enable dynamic adjustments in structure based on usage patterns, enhancing performance in real-time applications.
Quantum computing also promises a paradigm shift. Traditional data structures may not suffice; new designs that leverage quantum principles could emerge, dramatically speeding up processing times.
Moreover, as big data continues to grow, there’s an increasing demand for scalable solutions. Future developments might focus on hybrid models that combine different types of structures for optimal versatility and efficiency.
Cloud computing will influence how we design and implement these systems. Data accessibility and distribution across networks can lead to innovative approaches tailored for decentralized architectures.
Conclusion: The Importance of Understanding and Utilizing
Understanding data structures is crucial for anyone involved in programming or software development. They serve as the building blocks of efficient algorithms and applications.
When you grasp the various types of data structures, such as arrays, linked lists, stacks, and queues, you’re better equipped to choose the right one for your specific needs. Each structure offers unique advantages that can optimize performance and resource management.
Knowledge about these constructs not only enhances coding skills but also improves problem-solving capabilities. As technology evolves and new challenges arise, being well-versed in data structures ensures you remain adaptable and innovative.
Furthermore, real-world applications span across industries—from managing databases to powering complex software systems. The ability to implement effective data structures will set you apart in a competitive job market.
As future developments emerge within this field, staying informed will provide opportunities for growth and advancement. Embracing the importance of understanding and utilizing data structures opens doors to endless possibilities in technology today.